The LongListSelector is great for long lists in Windows Phone 7, but getting your data into the right format can be tricky and confusing (certainly took me a while to work out what to do!). Especially if you want to use ‘pure’ binding to an ObservableCollection.
The answer that I found easiest to understand and follow was to use ‘nested Observable collections’. This also ensure that things are kept up-to-date in the UI without additional coding.
To help me understand what was need I broke it down into some simple stages. I am sure it can be done with a single LINQ statement.
Let say you have a list of contacts that you would like to display with the typical A-Z short cuts as shown here. This includes showing group titles that contain no child records too!
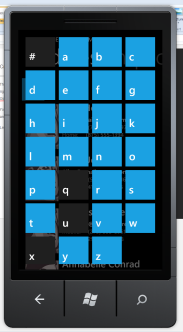
Create the basic object – Contacts
We’ll start with the simple Contact class that has two 3 properties. One is derived to create the key value. This can be any value, but here it’s the first letter of the Name value in lower case. If your data is changing then you should implement the INotifyPropertyChanged interface. I have left it out here for simplicity.
namespace PhoneTester1.Model
{
public class Contact
{
public string Name { get; set; }
public string JobTitle { get; set; }
private string _NameKey;
/// <summary>
/// First letter of the Name. Used for grouping in the long list selector
/// </summary>
public string NameKey
{
get
{
//If not set then
if (_NameKey == null)
{
//get the first letter of the Name
char key = char.ToLower(this.Name[0]);
if (key < 'a' || key > 'z')
{
key = '#';
}
_NameKey = key.ToString();
}
return _NameKey;
}
}
}
}
Create the Group Observable Collection
Then we’ll create a Group class. I have called it GroupOC for Grouping Observable Collections.
using System.Collections.ObjectModel;
namespace PhoneTester1.Model
{
public class GroupedOC<T>:ObservableCollection<T>
{
/// <summary>
/// The Group Title
/// </summary>
public string Title
{
get;
set;
}
/// <summary>
/// Constructor ensure that a Group Title is included
/// </summary>
/// <param name="name">string to be used as the Group Title</param>
public GroupedOC(string name)
{
this.Title = name;
}
/// <summary>
/// Returns true if the group has a count more than zero
/// </summary>
public bool HasItems
{
get
{
return (Count != 0);
}
private set
{
}
}
}
}
Create the Helper method to Group the Contacts
I created a static method called CreateGroupedOC in a static Collection Helper class called CollectionHelper. This can then be called on to group everything up.
using System.Collections.ObjectModel;
using System.Linq;
using PhoneTester1.Model;
namespace PhoneTester1.Functions
{
public static class CollectionHelpers
{
/// <summary>
/// Groups a passed Contacts ObservableCollection
/// </summary>
/// <param name="InitialContactsList">Unordered collection of Contacts</param>
/// <returns>Grouped Observable Collection of Contacts suitable for the LongListSelector</returns>
public static ObservableCollection<GroupedOC<Contact>> CreateGroupedOC(ObservableCollection<Contact> InitialContactsList)
{
//Initialise the Grouped OC to populate and return
ObservableCollection<GroupedOC<Contact>> GroupedContacts = new ObservableCollection<GroupedOC<Contact>>();
//first sort our contacts collection into a temp List using LINQ
var SortedList = (from con in InitialContactsList
orderby con.Name
select con).ToList();
//Now enumerate throw the alphabet creating empty groups objects
//This ensure that the whole alphabet exists even if we never populate them
string Alpha = "#abcdefghijklmnopqrstuvwxyz";
foreach (char c in Alpha)
{
//Create GroupedOC for given letter
GroupedOC<Contact> thisGOC = new GroupedOC<Contact>(c.ToString());
//Create a temp list with the appropriate Contacts that have this NameKey
var SubsetOfCons = (from con in SortedList
where con.NameKey == c.ToString()
select con).ToList<Contact>();
//Populate the GroupedOC
foreach (Contact csm in SubsetOfCons)
{
thisGOC.Add(csm);
}
//Add this GroupedOC to the observable collection that is being returned
// and the LongListSelector can be bound to.
GroupedContacts.Add(thisGOC);
}
return GroupedContacts;
}
}
}
Tie it together in your ViewModel
In my projects I am usually populating a collection of objects either from a database or web service. Here we’ll start with a collection of Contacts that is populated in a random order. This ObservableCollection we would pass to the method shown above (CreateGroupedOC ) to group it and return a grouped ObservableCollection.. This can then be referenced in your ViewModel and bound to the LongListSelector control.
I also use the very handy MVVM Light Toolkit produced by the very excellent Laurent Bugnion of GalaSoft. This you can find at http://mvvmlight.codeplex.com/ and some info about it at http://www.galasoft.ch/mvvm/getstarted/. I strongly recommend using it.
I have included my whole ViewModel below. Hopefully the comments make it self explanatory!
using System;
using System.Collections.ObjectModel;
using System.Text;
using GalaSoft.MvvmLight;
using PhoneTester1.Functions;
using PhoneTester1.Model;
namespace PhoneTester1.ViewModel
{
/// <summary>
/// This class contains properties that the main View can data bind to.
/// You can also use Blend to data bind with the tool's support.
/// This code is provided as is by Hard Medium Soft Ltd.
/// see us at http://www.hardmediumsoft.com
/// </summary>
public class MainViewModel : ViewModelBase
{
public string ApplicationTitle
{
get
{
return "Nicholas Rogoff for Hard Medium Soft Ltd.";
}
}
public string PageName
{
get
{
return "Tester App";
}
}
/// <summary>
/// The <see cref="GroupedContacts" /> property's name.
/// </summary>
public const string GroupedContactsPropertyName = "GroupedContacts";
private ObservableCollection<GroupedOC<Contact>> _GroupedContacts = new ObservableCollection<GroupedOC<Contact>>();
/// <summary>
/// Gets the GroupedContacts property.
///
/// Changes to that property's value raise the PropertyChanged event.
/// This property's value is broadcasted by the Messenger's default instance when it changes.
/// </summary>
public ObservableCollection<GroupedOC<Contact>> GroupedContacts
{
get
{
return _GroupedContacts;
}
set
{
if (_GroupedContacts == value)
{
return;
}
var oldValue = _GroupedContacts;
_GroupedContacts = value;
// Update bindings, no broadcast
RaisePropertyChanged(GroupedContactsPropertyName);
}
}
/// <summary>
/// Initializes a new instance of the MainViewModel class.
/// </summary>
public MainViewModel()
{
//Populate some Contacts with random strings
ObservableCollection<Contact> tempContactsOC = new ObservableCollection<Contact>();
for (int i = 0; i < 50; i++)
{
Contact tempContact=new Contact(){Name = RandomString(12,false,i) + " - " + i, JobTitle=RandomString(18,false,i)};
tempContactsOC.Add(tempContact);
}
//Create our Nested ObservableCollection
GroupedContacts = CollectionHelpers.CreateGroupedOC(tempContactsOC);
}
#region Random String generator
/// <summary>
/// Generates a random string with the given length
/// </summary>
/// <param name="size">Size of the string</param>
/// <param name="lowerCase">If true, generate lowercase string</param>
/// <returns>Random string</returns>
private string RandomString(int size, bool lowerCase, int seed)
{
StringBuilder builder = new StringBuilder();
Random random = new Random((int)DateTime.Now.Ticks * seed);
char ch;
for (int i = 0; i < size; i++)
{
ch = Convert.ToChar(Convert.ToInt32(Math.Floor(26 * random.NextDouble() + 65)));
builder.Append(ch);
}
if (lowerCase)
return builder.ToString().ToLower();
return builder.ToString();
}
#endregion
}
}
If you think you can make this simpler or better please let me know. Thanks.
Like this:
Like Loading...